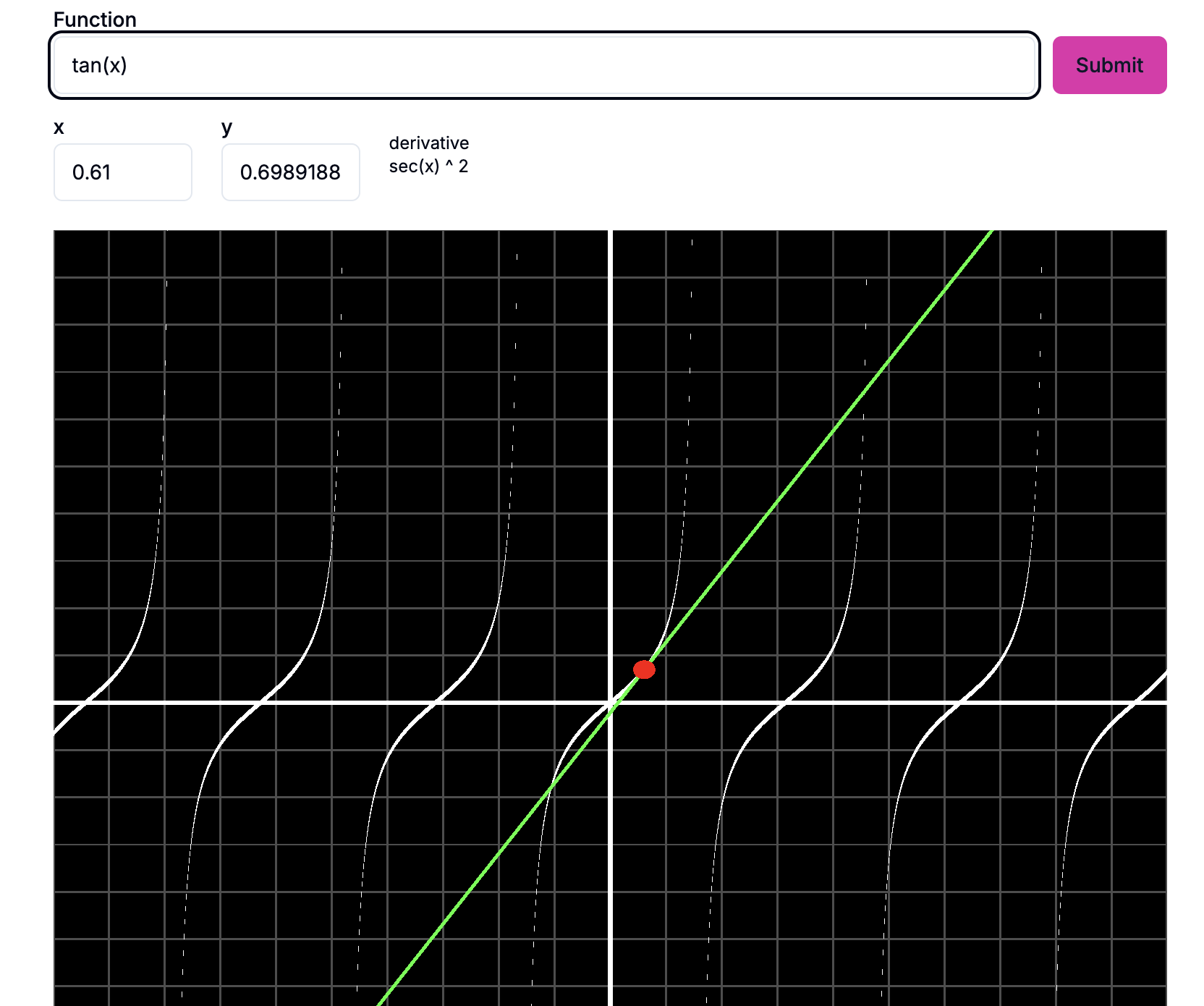
Graphing Calculator
I rewrote an old project of mine (https://github.com/jzohdi/simple_graph) to use webgl shaders to further my knowledge of webgl rendering.
To make this work, I built glsl_math —a tiny compiler that takes user‑entered calculus expressions and turns them into GLSL code you can run in a fragment shader. See tests for more examples here.
At a high level this experiment:
- Parses the user’s formula (e.g.
sin(x) + cos(x)
orx^2 + 2*x + 1
). - Transpiles it via glsl_math into a glsl compatible snippet.
- Injects that snippet into a fragment shade (compile shader program at runtime)
- Renders the function over a 2D viewport by drawing a full‑screen quad and coloring pixels according to their distance from the curve (plus axes, gridlines, and optional tangents).
In the future I would like to support rendering multiple functions at the same time.
Supported Math Functionality for Transpilation
- Variables:
x
- Integer literals:
2
- Floating‑point literals:
2.1
- Constants:
PI
/Pi
/pi
→3.14159265359
,E
/e
→2.71828182846
- Addition:
a + b
- Subtraction:
a - b
- Multiplication:
a * b
- Division:
a / b
- Exponentiation:
x^y
→pow(x, y)
in GLSL - Parentheses:
( … )
- Trigonometric:
sin(x)
,cos(x)
,tan(x)
,asin(x)
,acos(x)
,atan(x)
- Logarithms:
log(x)
(natural log) - Roots & absolutes:
sqrt(x)
,abs(x)
- Rounding:
floor(x)
,ceil(x)